Software architecture refers to the way a software system is organized. This organization includes all components, how they interact, the environment in which they operate, and the principles used to design the software. In many cases, it can also include the evolution of the software into the future.
Introduce software architectures
Hexagonal architecture
Hexagonal architecture (also known as Ports and Adapters architecture) is a type of software architecture that can be benchmarked alongside other software architectures. It is based on isolating the core business logic from outside concerns by separating the application into loosely coupled components.
Instead of tightly coupling our use case class (or service class) to an external REST API, we can introduce a Port. This Port defines the interface that the use case class needs to implement to fetch data, independently of any specific API standard or database. Then, we can create an Adapter that implements the Port interface and delegates the calls to the external API or another external service. This approach contributes to a decoupled design and provides a consistent basis to benchmark the software architectures based on testability and maintainability.
The concept of clean architecture
Robert C. Martin defined the concept of clean architecture. In this architecture, developers can divide systems into two main elements: the policies and the details. The policies are the business rules and procedures, and the details are the items necessary to carry out the policies. Developers can defer the choice of database or framework during the initial stages of development, as these are merely implementation details that do not influence the core policies and can be modified later if needed. This separation of concerns enhances flexibility and makes it easier to benchmark the software architectures based on adaptability to change.
In Clean Architecture there is a well-defined division of layers. Architects or developers must divide the system into four layers: the domain layer, the application layer, the infrastructure layer, and the frameworks & drivers layer.
- Domain layer: This Layer is responsible for the business logic of the application. It is the most stable layer and essentially represents the heart of the application. Its consistency is crucial when benchmarking software architectures for maintainability and business rule isolation.
- Application layer: This layer contains the application-specific business rules. The application layer implements all the use cases of the application. It also utilizes the domain classes. However, it remains isolated from the implementation details of outer layers, such as databases, adapters, and other infrastructure components. Clear separation enables modular design, essential when benchmarking software architectures for maintainability and scalability.
- Infrastructure layer: This layer holds all concrete implementations, such as repositories, adapters, and database connections.
- Developers build the Frameworks & Drivers layer with tools like databases and frameworks, impacting architecture benchmarking.
Flux
Flux is an application architecture that Facebook uses internally for building a client-side web application with React. This is not a library or a framework. Instead, it is a form of architecture that complements React as the view layer and follows the Unidirectional Data Flow model. It is particularly effective for projects with dynamic data. This approach helps ensure the data remains consistently updated. By maintaining a predictable flow of information, developers can manage state changes more efficiently. As a result, developers can significantly reduce the likelihood of runtime errors.
Flux applications have three major components in dealing with data:
- Store: The store manages the state. A well-designed store can manage both the domain state and the user interface state in a centralized and consistent manner. This capability is particularly important when we benchmark the software architectures for state coherence, maintainability, and UI responsiveness. A store manages multiple objects. It is the single source of truth in regard to those specific objects. In an application, there can be many stores. For example, in a well-structured application, separate stores such as BookStore, AuthorStore, and UserStore can be created to manage distinct parts of the application’s state. This modularization enhances clarity and maintainability, which are essential when we benchmark the software architectures in terms of scalability and state management efficiency.
- Dispatcher: Dispatcher is a single object that broadcasts actions/events to all registered stores. Stores need to register for events when the application starts. When an action comes in, it will pass that action to all registered stores.
- View: View is the user interface component. It is responsible for rendering the user interface and for handling the user interaction. Views are in a tree structure.
- Actions: An action is a plain object that contains all the information necessary to do that action. Actions have a type property identifying the action type.
- Action creators: The practice is to encapsulate the code, creating actions in functions. Developers refer to the functions that create and dispatch actions as action creators.
The Model-View-Controller (MVC)
The Model-View-Controller (MVC) architecture separates an application into three main logical components Model, View, and Controller. Developers build each architectural component to handle specific aspects of application development. It isolates the business logic and presentation layer from each other.
The MVC architecture has 3 components:
- Controller: The controller is the component that enables the interconnection between the views and the model so it acts as an intermediary. The controller doesn’t have to worry about handling data logic, it just tells the model what to do
- View: Developers use the View component to implement all the UI logic of the application. It generates a user interface for the user. The controller retrieves data from the model component and passes it to the view, which then uses this data to create the user interface.
- Model: The Model component corresponds to all the data-related logic that the user works with. Developers can use this to represent either the data transferred between the View and Controller components or any other data related to business logic. It can add or retrieve data from the database.
MVVM (Model-View-ViewModel) architecture
MVVM (Model-View-ViewModel) architecture cleanly separates the business logic of an application from the user interface. The ultimate goal of MVVM architecture is to make the view completely independent from the application logic.
The MVVM architecture has 3 components:
- Model: The model represents the app’s domain model, which can include a data model as well as business and validation logic. It communicates with the ViewModel and lacks awareness of the View.
- View: The View represents the user interface of the application and holds limited, purely presentational logic that implements visual behavior. The View is completely agnostic to the business logic. In other words, the View never contains data, nor manipulates it directly. It communicates with the ViewModel through data binding and is unaware of the Model.
- ViewModel: The ViewModel is the link between the View and the Model. It implements and exposes public properties and commands that the View uses by way of data binding. If any state changes occur, the ViewModel notifies the View through notification events.
Analyze the advantages and disadvantages of architectures
Hexagonal Architecture
Advantages | Disadvantages |
– Highly flexible which allows for easy adaptation to changing requirements and business needs- Highly testable due to its modular design- Separation of concerns makes it highly scalable- Easy to switch between different frameworks, libraries, or databases- Easy to maintain and update the system, reducing the risk of bugs or errors. | – More complex to apply hexagonal architecture for small projects or teams with limited resources.- New developers may require a high learning curve.- Over-engineering is a risk with hexagonal architecture, it is possible to over-design the system, leading to unnecessary complexity and reduced maintainability |
Clean Architecture
Advantages | Disadvantages |
– Separating business logic from infrastructure makes clean architecture easier to modify or replace specific components. – More comprehensive testing of individual components and their interactions- Highly flexible and adaptable to change business needs or technological advancements- Higher maintainability and reduced technical debt- Independent of any particular framework or technology, making it easier to switch between different tools or platforms | – More complex to apply clean architecture for small projects or teams with limited resources.- New developers may require a high learning curve.- Over-engineering is a risk with hexagonal architecture, it is possible to over-design the system, leading to unnecessary complexity and reduced maintainability |
Flux Architecture
Advantages | Disadvantages |
---|---|
– Provides a single source of truth, which means that all data is stored in a central store and changes can only be made through predefined actions. – It uses unidirectional data flow, which makes it easier to understand how data is processed throughout the application.- Separates data storage and state management from view components- More comprehensive testing of individual components and their interactions | – More complex to apply flux architecture for small projects or teams with limited resources.- New developers may require a high learning curve.- Over-engineering is a risk with hexagonal architecture, it is possible to over-design the system, leading to unnecessary complexity and reduced maintainability. |
MVC Architecture
Advantages | Disadvantages |
– Separates the application logic into three distinct components – Model, View, and Controller, which helps to achieve a clean and organized code structure- Easy to reuse the code components across multiple projects- Highly maintainable due to the separation of concerns, which makes it easier to modify, test, and debug the application- Easy to learn and implement, which makes it a popular choice for beginners- Easy to scalable: addition of new features or modifications without affecting the existing codebase | – Can become complex in larger applications with many components and interactions- May lead to tight coupling between the components, which can reduce flexibility and increase dependencies- Possible to have duplicate code across different components in MVC architecture, leading to a less efficient codebase |
MVVM Architecture
Advantages | Disadvantages |
– Separates the user interface (View) from the data and business logic (Model) and new component (ViewModel) which leads to a clean and organized code structure.- Data bindings in MVVM architecture make it easier to test the application logic in isolation- Easy to reuse the code components across multiple projects- Allows for a high degree of flexibility, as the ViewModel can be customized for different views, and the model can be changed without affecting the view. | – Can become complex in larger applications with many components and interactions- New developers may require a high learning curve- It is possible to over-design the system leading to unnecessary complexity and reduced maintainability- Data binding is declarative, it can be harder to debug than traditional, imperative code. |
The case use of the above software architecture
- Hexagonal architecture is a good fit for any application that has complex business logic and multiple layers of data access. An example of a use case to use hexagonal architecture is an SMS notification system that we need to include in our application. We want to use a third-party notification system in order to accomplish our business goals. Integrating such external services also plays a role when we benchmark the software architectures, particularly in terms of extensibility and external dependency management.
- Clean architecture is a good fit for large-scale applications with complex business logic, multiple teams working on the same codebase, or applications that are expected to evolve over time.
- Flux architecture can be applied to any type of application that requires unidirectional data flow and a predictable state management system. This architectural approach is especially useful for large-scale applications with complex user interfaces and data flows. It is therefore a great fit for projects that require real-time data synchronization, the ability to process large volumes of data, and a consistently stable user interface. These characteristics also become key factors when we benchmark the software architectures for responsiveness and reliability.
- MVC architecture is a good fit to manage the frontend and backend in smaller, separate components allowing for the application to be scalable, maintainable, and easy to expand.
- MVVM architecture is a good fit when we need to share a project with a designer and the design and development work can happen independently, we want more flexibility to change your views without having to refactor other logic in the code base.
In conclusion, software architecture is a critical component of software development, especially when building complex systems that require scalability, maintainability, and the integration of multiple technologies or systems. Understanding how to benchmark the software architectures helps teams evaluate and select the most appropriate architectural approach based on project requirements and future evolution. Depending on the needs and specific projects, different software architectures will apply.
Would you like to read more articles by Tekos’s Team? Everything’s here.
Reference
- https://www.sensedia.com/post/use-of-the-hexagonal-architecture-pattern
- https://embapro.com/frontpage/swotcoanalysis/28975-hexagon-composites
- https://bitloops.com/docs/bitloops-language/learning/software-architecture/hexagonal-architecture
- https://dev.to/dyarleniber/hexagonal-architecture-and-clean-architecture-with-examples-48oi
- https://www.castsoftware.com/glossary/what-is-software-architecture-tools-design-definition-explanation-best
- https://www.geeksforgeeks.org/mvc-framework-introduction
- https://www.freecodecamp.org/news/an-introduction-to-the-flux-architectural-pattern-674ea74775c9
- https://www.makeuseof.com/mvc-mvp-mvvm-which-choose
Author
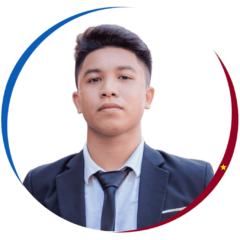