Introduction
Recently, while working on a core project and juggling multiple others, I gained a deeper appreciation for the critical role Symfony bundles play in efficient development. Creating these bundles not only enhances code reusability but also paves the way for a smarter, faster, and more organized approach to managing future projects. In this article, I want to introduce the basics of Symfony bundles and highlight the significance and challenges of bundles.
1. Bundle
1.1 What is the bundle?
A bundle is a structured package of functionality that can be reused across different applications. Bundles allow you to organize and encapsulate code, making it modular and easier to manage
1.2 Why do we need a bundle?
Bundle offers several advantages that contribute to the framework’s flexibility and efficiency. Here are some reasons why bundles are important:
– Modularity:
Bundles allow you to break down your application into smaller, manageable components. This modularity makes developing, maintaining, and scaling your application easier.
– Reusability
Bundles can be reused across multiple projects. When you create a bundle encapsulating a specific feature, it becomes straightforward to integrate it into applications seamlessly, eliminating the need to rewrite code.
– Encapsulation
The bundle encapsulates its configuration, routing, services, controllers, templates, and assets. This keeps related code together, making it easier to understand and manage. This approach also plays a crucial role in isolating functionality, ensuring that modifications within one bundle remain independent and do not impact others.
– Separation of Concerns
Using bundles encourages a separation of concerns within your application. Each bundle can focus on a specific aspect of your application leading to cleaner and more organized code.
– Scalability
As your application grows, bundles make it easier to manage complexity. You can develop and test bundles independently, allowing teams to work on different features without conflicts.
– Customizability
Bundles can be enabled or disabled in your application’s configuration. This level of flexibility enables you to tailor your application’s features precisely to align with your unique needs and requirements.
– Easy Integration
Bundles can easily integrate with Symfony’s core features and other bundles. This integration simplifies the process of adding functionality to your application.
1.3 Symfony bundles
Here are these common bundles of Symfony
1.3.1. Core Symfony Bundles
+ FrameworkBundle: provides the basic functionality of the Symfony framework, including routing, templating, and configuration management
+ SecurityBundle: manages authentication, authorization, and security features for your application.
+ TwigBundle: integrates the Twig templating engine, allowing for easy rendering of templates within Symfony applications.
+ MonologBundle: integrates the Monolog logging library, allowing for flexible logging configurations and support for various log handlers
+DoctrineBundle: provides integration with Doctrine ORM, enabling database interactions through an object-oriented approach.
1.3.2 User Management and Authentication
+ FOSUserBundle: manage user registration, login, and profile management.
+ LexikJWTAuthenticationBundle: provides JWT (JSON Web Token) authentication support for APIs
1.3.3 Caching and Performance
+ DoctrineCacheBundle: integrates caching capabilities into your application using Doctrine’s caching features
+ SymfonyCacheBundle: provides a unified caching interface, compatible with various caching backends (e.g., Redis, Memcached).
…..
Other Useful Bundles (ApiPlatform, NelmioApiDocBundle,….)
2. How to use
2.1 Symfony bundle
Sample how to use a monolog bundle of Symfony with simple steps:
Frist: Install by using the composer
#bash
composer require symfony/monolog-bundle
Second: configure Monolog by creating config/packages/monolog.yaml file
#yaml
monolog:
handlers:
main:
type: stream
path: "%kernel.logs_dir%/%kernel.environment%.log"
level: debug
Finally: use in your code, sample: TestController.php
namespace App\Controller;
use Psr\Log\LoggerInterface;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
class TestController extends AbstractController
{
private $logger;
public function __construct(LoggerInterface $logger)
{
$this->logger = $logger;
}
public function index(): Response
{
$this->logger->info('This is an info message.');
$this->logger->error('This is an error message.');
return new Response('Check the logs!');
}
}
2.2 Create new bundle:
Creating a new bundle in Symfony involves setting up a specific directory structure
src/
└── TekosInteractive/
└── YourBundle/
├── DependencyInjection/
│ ├── Configuration.php
│ └── YourBundleExtension.php
├── Resources/
│ ├── config/
│ │ └── services.yaml
│ └── views/
├── YourBundle.php
└── Controller/
Or create new bundle with ADR structure (ADR – Action-Domain-Responder)
src/
└── TekosInteractive/
└── YourBundle/
├── Action/
├── Domain/
├── Infrastructure/
├── DependencyInjection/
│ ├── Configuration.php
│ └── TekosInteractiveYourExtension.php
├── Resources/
│ ├── config/
│ │ └── services.yaml
└── TekosInteractiveYourBundle.php
3. Challenges and difficulties associated with using your bundle
3.1 Complexity in Configuration
If you are using your own bundle (a newly created one), ensure that it adheres to the correct structure; otherwise, it may not function as expected or could behave unpredictably. Additionally, if your bundle requires a specific database, make sure it includes a migration with an appropriate namespace (e.g., UserBundleMigrations).
namespace UserBundleMigrations;
use Doctrine\DBAL\Schema\Schema;
use Doctrine\Migrations\AbstractMigration;
final class Version20241007100101 extends AbstractMigration
{
}
In your application need more specific config doctrine.yaml
doctrine:
dbal:
url: '%env(resolve:DATABASE_URL)%'
orm:
auto_generate_proxy_classes: true
enable_lazy_ghost_objects: true
naming_strategy: doctrine.orm.naming_strategy.underscore_number_aware
auto_mapping: true
mappings:
App:
is_bundle: false
type: 'yml'
dir: '%kernel.project_dir%/config/doctrine'
prefix: 'App\Domain\Entities'
alias: App
TekosInteractiveSaaUserBundle:
is_bundle: true
type: 'yml'
dir: 'config/doctrine'
prefix: 'TekosInteractive\SaaUserBundle\Domain\Entities'
alias: TekosInteractiveSaaUserBundle
Therefore, when you run your application, it will execute migrations for both your application and your bundle.
3.2. Version Compatibility:
When creating a bundle, ensuring version compatibility is crucial to maintaining the functionality and stability of your application.
Besides that, we should think about the version
Semantic Versioning: Follow semantic versioning (SemVer) principles. Your version numbers should indicate the type of changes:
Major version: Incompatible API changes.
Minor version: Backward-compatible functionality added.
Patch version: Backward-compatible bug fixes.
3.3 Namespace Conflicts
When creating a new Symfony bundle, managing namespace conflicts is essential to avoid issues with autoloading, class resolution, and code maintainability.
Here are strategies to effectively handle namespace conflicts:
+ Use a Unique Namespace: follow a Naming Convention, A common practice is to use a top-level namespace that includes your vendor name, followed by the bundle name. For example namespace TekosInteractive\SaaUserBundle;
.+ Use Autoloading Standards
Composer Autoloading: Ensure your bundle is properly set up for autoloading with Composer. Define your namespace in the composer.json file:
{
"autoload": {
"psr-4": {
"TekosInteractive\\SaaUserBundle\\": "src/"
}
}
}
3.4. Debugging Difficulties
Here are some common debugging difficulties and strategies to effectively troubleshoot them:
– Autoloading Issues like classes not found or not autoloaded correctly. So we will check the namespace and directory structure, and ensure that your namespaces match your directory structure as defined in your composer.json file.
-Configuration Errors: incorrect service configuration leading to errors during dependency injection. So will check service definitions, check for deprecated or removed features, and then clean the cache
Conclusion
Overall, bundles are essential for building robust, maintainable, and scalable applications in Symfony. They help you organize your code effectively, promote best practices, and enable the reuse of functionality—making development faster and more efficient.
To use the available bundle is simple, you just need to follow the instructions closely, but to build a new bundle, you need to have in-depth knowledge.
Would you like to read more articles by Tekos’s Team? Everything’s here.
References
- https://symfony.com/doc/current/bundles.html
- https://medium.com/symfony-mastery/how-to-create-a-bundle-in-symfony-18bf584835d1
- https://symfony.com/doc/current/bundles/best_practices.html
Author
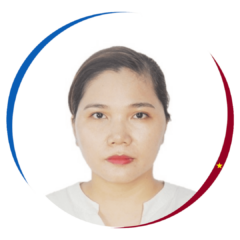